效果
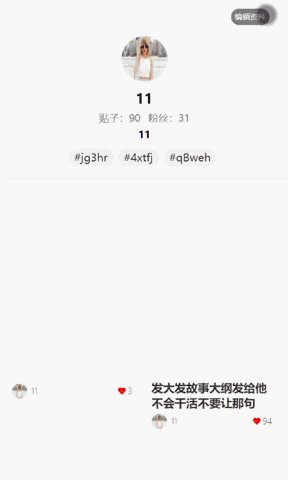
代码
editInfo.js
javascript">
Page({
data: {
dataList: []
},
chooseWxImage: function () {
const that = this;
wx.chooseImage({
count: 1,
sizeType: ['original', 'compressed'],
sourceType: ['album', 'camera'],
success: function (res) {
wx.showToast({
title: '正在上传...',
icon: 'loading',
mask: true,
duration: 10000
});
console.log(res);
console.log(res.tempFilePaths[0]);
that.upImgs(res.tempFilePaths[0], 0);
},
fail: function (res) {
console.log('fail', res);
},
complete: function (res) {
}
});
},
upImgs: function (imgurl, index) {
const that = this;
wx.uploadFile({
url: `http://api`,
filePath: imgurl,
name: 'file',
header: {
'content-type': 'multipart/form-data'
},
formData: {},
success: function (res) {
const datas = JSON.parse(res.data);
console.log(datas);
if (datas.code === 1) {
wx.setStorageSync('PROFILEURL', 'http://' + datas.data);
const profileUrl = wx.getStorageSync('PROFILEURL');
that.setData({
profileUrl: profileUrl
});
wx.showToast({
title: '头像上传成功',
icon: 'success',
duration: 2000,
mask: true,
success: res => {}
});
console.log('success', res);
} else {
wx.showModal({
title: '错误提示',
content: datas.message,
showCancel: false,
success: function (res) { }
});
console.log('fail', datas.message);
}
},
fail: function (res) {
console.log('fail', res);
wx.hideToast();
wx.showModal({
title: '错误提示',
content: '上传图片失败',
showCancel: false,
success: function (res) { }
});
}
});
},
onLoad: function (options) {
},
onReady: function () {
},
onShow: function () {
},
onHide: function () {
},
onUnload: function () {
},
onPullDownRefresh: function () {
},
onReachBottom: function () {
},
onShareAppMessage: function () {
}
});
profile.js
javascript">
const USERID = wx.getStorageSync('USERID');
const ROLEID = wx.getStorageSync('ROLEID');
const PROFILEURL = wx.getStorageSync('PROFILEURL');
const NICKNAME = wx.getStorageSync('NICKNAME');
const POSTNUM = wx.getStorageSync('POSTNUM');
const FANSNUM = wx.getStorageSync('FANSNUM');
const INTRODUCE = wx.getStorageSync('INTRODUCE');
const TAGLIST = wx.getStorageSync('TAGLIST');
Page({
data: {
UserInfo: [{
profileUrl: PROFILEURL,
nickname: NICKNAME,
postNum: POSTNUM,
fansNum: FANSNUM,
introduce: INTRODUCE,
tagList: TAGLIST
}],
pageNum: 1,
userPageSize: 4,
personalId: USERID,
userId: USERID,
onRefresh: false,
roleId: ROLEID
},
updateUserInfo: function () {
const PROFILEURL = wx.getStorageSync('PROFILEURL');
const NICKNAME = wx.getStorageSync('NICKNAME');
const POSTNUM = wx.getStorageSync('POSTNUM');
const FANSNUM = wx.getStorageSync('FANSNUM');
const INTRODUCE = wx.getStorageSync('INTRODUCE');
const TAGLIST = wx.getStorageSync('TAGLIST');
const that = this;
for (let i = 0; i < that.data.UserInfo.length; i++) {
const profileUrl = 'UserInfo[' + i + '].profileUrl';
const nickname = 'UserInfo[' + i + '].nickname';
const postNum = 'UserInfo[' + i + '].postNum';
const fansNum = 'UserInfo[' + i + '].fansNum';
const introduce = 'UserInfo[' + i + '].introduce';
const tagList = 'UserInfo[' + i + '].tagList';
that.setData({
[profileUrl]: PROFILEURL,
[nickname]: NICKNAME,
[postNum]: POSTNUM,
[fansNum]: FANSNUM,
[introduce]: INTRODUCE,
[tagList]: TAGLIST
});
}
},
gotoEditInfo: function (event) {
wx.navigateTo({
url: `../editInfo/editInfo?${this.data.dataList}`
});
},
onLoad: function (options) {
const that = this;
if (USERID === 0) {
wx.redirectTo({ url: 'pages/login/login' });
}
},
onReady: function () {
},
onShow: function () {
const that = this;
if (ROLEID === 1) {
that.updateUserInfo();
} else {
wx.showToast({
title: '用户未登录!',
icon: 'none',
duration: 2000,
mask: true,
success: res => {}
});
console.log('用户未登录!');
}
},
onHide: function () {
},
onUnload: function () {
},
onPullDownRefresh: function () {
},
onReachBottom: function () {
},
onShareAppMessage: function () {
}
});